Static blogs with complicated build tools
2025-02-11
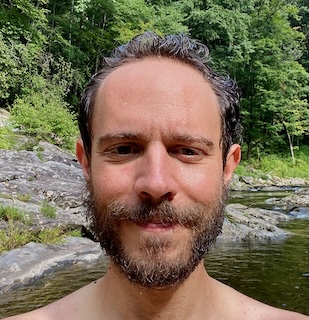
Occasionally, I come across some cool blogs with a very approachable design. No ads popping up on every side of the screen, no weird scrolling behavior, maybe a monospace font. They invite you to read what they have to say in a anxiety reducing manner. Maybe they’ll also have some retro color schemes that respect your devices light/dark theme preferences. Cool.
I don’t post on social media — its just not for me — but I do enjoy writing an essay here or there and thought it was time to throw something together. Wordpress would’ve been the quickest way — probably. But that’s no fun and I don't want 99% of the functionality. I like the idea of having a blog that can run with JavaScript disabled — no I dont run my browser with JavaScript disabled, but the appeal to simplicity is intriguing. So where does that land us? I like the Django templating system for simple stuff. I could piggy back of the MVC (MVT?) structure and build a dynamic blog and then just cache the whole site so it serves static… But Next.js is modern and I love to learn new things, so let’s build a static blog using Next.js!
Requirements
- Static site
- Design like a dynamic site
- Write blog posts in Markdown
- Theme switching (because that’s the responsible thing to do)
- SPA speed while also being a static site? (This wasn’t an original requirement but turned out to be why Next.js static exists)
Next.js Fundementals
This isn’t intended to be a Next.js tutorial but I’ll give a few thoughts on the framework. I prefer Vue over React but the React ecosystem is so big and mature that I’d rather use Next over Nuxt, go figure. The main feature of Next that makes this blog easy to design is the folder structure based routing. Throw some components in the pages/
folder and hit the ground running. The next feature is parameterized routes. Through a [slug].js
component in that pages folder and its now a dynamic route. Dynamic for the purpose of building but the end result is a static html file gets created for each path
. A path is generated by exporting getStaticPaths()
and returning and object that you’ll iterate over in your dynamic page:
export async function getStaticPaths() {
const postsDirectory = path.join(process.cwd(), 'posts');
const filenames = fs.readdirSync(postsDirectory);
const paths = filenames.map((filename) => ({
params: {
slug: filename.replace(/\.md$/, ''),
},
}));
return {
paths,
fallback: false,
};
}
This all happens at build time, despite being a part of a react app. In the above example, I build each of my blog post pages where the path is the file name without the extension. Weird at first, but I kind of like it.
In a similar concept, the actual content of the pages are passed as props by exporting getStaticProps()
. This is where I parse my Markdown files, pull out the meta data, format the Markdown into html (notice the parameter is the output from a path above):
export async function getStaticProps({ params }) {
const postsDirectory = path.join(process.cwd(), "posts");
const filePath = path.join(postsDirectory, `${params.slug}.md`);
const fileContents = fs.readFileSync(filePath, "utf8");
const { data, content } = matter(fileContents);
const processedContent = await remark().use(html).process(content);
const contentHtml = processedContent.toString();
return {
props: {
post: {
...data,
content: contentHtml
}
}
}
}
Theming
I’ve seen some blogs have light/dark mode switch — I always thought that was a nice touch. So I wanted a few things though:
- By default, the theme will respect the device mode
- This should work with JavaScript enabled and with is disabled (its a static site after all)
- User should be able to easily change that mode and it should stay that way
- Switching to your devices default mode should make the site start to mirror your device setting again
I’m not 100% sure this leads to the best UX, but I think it’s pretty close. The other option would be to make a second button that reverts to default.
Anyway, I made this all happen, but it’s not pretty. The theme is css variables on :root
with two additional declarations: one media query with the rule prefers-color-scheme: light
to handle no JS. and then another duplicate rule with the attribute [data-theme='light']
. The way it works is I inject some code right at the beginning of the html that will delete the prefers-color-scheme rule right away if it exists. If JS is disabled, this doesnt occur and the css just works as intended. If JS is enabled, the rule is deleted, then I check local storage for a previously set theme, if found, set the data-theme attribute, if not found, grab the device default theme and set that to data-theme attribute. Then I have a little switch that will set the (or delete) the localstorage theme while also toggling the data-theme attribute. All in all, it works as intended.
Here’s my theme:
:root {
--background: #282a36;
--current-line: #44475a;
--foreground: #f8f8f2;
--comment: #6272a4;
--cyan: #8be9fd;
--green: #50fa7b;
--orange: #ffb86c;
--pink: #ff79c6;
--purple: #bd93f9;
--red: #ff5555;
--yellow: #f1fa8c;
--background-contrast: #373844;
}
@media (prefers-color-scheme: light) {
:root {
--background: #f8f8f2;
--current-line: #e0e0e0;
--foreground: #282a36;
--comment: #6272a4;
--cyan: #007acc;
--green: #228b22;
--orange: #ff8c00;
--pink: #d81b60;
--purple: #6a1b9a;
--red: #d32f2f;
--yellow: #fbc02d;
--background-contrast: #e0e0e6;
}
}
/* Switch to light version of theme if the user prefers light mode */
[data-theme="light"] {
--background: #f8f8f2; /* Light background */
--current-line: #e0e0e0; /* Light gray for current line */
--foreground: #282a36; /* Dark gray for text */
--comment: #6272a4; /* Muted blue for comments */
--cyan: #007acc; /* Bright blue for accents */
--green: #228b22; /* Forest green for accents */
--orange: #ff8c00; /* Bright orange for accents */
--pink: #d81b60; /* Bright magenta for accents */
--purple: #6a1b9a; /* Medium purple for accents */
--red: #d32f2f; /* Bright red for accents */
--yellow: #fbc02d; /* Bright yellow for accents */
--background-contrast: #e0e0e6; /* Light gray for contrast */
}